Write your first MPxCommand (Maya Python API)
- Dilen Shah
- Aug 18, 2021
- 3 min read
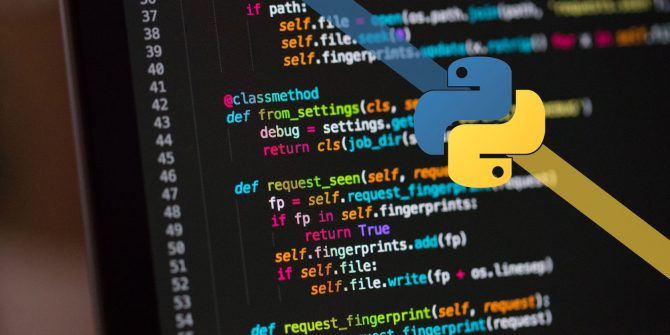
Let's learn how to write a Command in the Maya Python API using HelloWorld example, we will split this into 2 different posts which will introduce this idea of extending Maya by implementing a new command and a new node. If you're not familiar with hello world examples they are typically very simple pieces of code used to illustrate the basics of a programming language, in this case, I will be using the examples to demonstrate the basics of creating commands in nodes and how to register and the register them with Maya.
This post will focus on writing an MPxCommand, the example for this lesson will add a new HelloWorld command to Maya that when called will print out Hello World in the output window.
Let's first talk about the template that will be used pretty much every time to write a new Command, Node, Deformer for Maya using the API. Here is a code snippet you can use as a starting point.
import maya.api.OpenMaya as om import maya.cmds as cmds def maya_useNewAPI(): """ Tell Maya this plugin uses the Python API 2.0. """ pass def initializePlugin(plugin): """ """ vendor = "Dilen Shah" version = "1.0.0" om.MFnPlugin(plugin, vendor, version) def uninitializePlugin(plugin): """ """
pass
This file should always be saved to a location on your Maya plugin path so Maya is aware of it and can load it. To begin I need to create a new class for my Hello World command and this will extend the Maya API's MPXCommand class which is the base class for all commands.
To initialize the plug-in I'm going to create a new class, this is going to be called HelloWorldCmd and this extends the empty MPxCommand class found in your OpenMaya package.
class HelloWorldCmd(om.MPxCommand):
COMMAND_NAME = "HelloWorld"
def __init__(self): """ """ super(HelloWorldCmd, self).__init__() def doIt(self, args): """ Called when the command is executed in script """ print("My First MPxCommand, Hello World!") @classmethod def creator(cls): """ Returns an instance of the HelloWorldCmd """ return HelloWorldCmd()
COMMAND_NAME, is used to specify what the command will be called in Maya, for example, the select or polySphere commands that already exist in Maya.
For the init method, I am just going to call the super method, so we can call the MPxCommands methods.
For the doIt method, this is a required method for all commands. Now when someone executes the command through script Maya calls the commands doIt method. This is where all of the logic for the command is implemented because this command is only going to print "My First MPxCommand, Hello World!" to the output window I simply need to add a print function.
Lastly, the creator method is provided to Maya when the command is registered and used to get an instance of this command. This method is going to be a class method, and I'm going to name it creator, and all this method does is return a new instance, of the Hello World command.
All that is left to do now is register this command with Maya in the initializePlugin function, add the matching call to de-register it in the uninitializePlugin function.
def initializePlugin(plugin): """ Entry point for a plugin """ vendor = "Dilen Shah" version = "1.0.0" plugin_fn = om.MFnPlugin(plugin, vendor, version) try: plugin_fn.registerCommand(HelloWorldCmd.COMMAND_NAME, HelloWorldCmd.creator) except: om.MGlobal.displayError("Failed to register command: {0}".format(HelloWorldCmd)) def uninitializePlugin(plugin): """ Exit point for a plugin """ plugin_fn = om.MFnPlugin(plugin)
try: plugin_fn.deregisterCommand(HelloWorldCmd.COMMAND_NAME) except: om.MGlobal.displayError("Failed to deregister command: {0}".format(HelloWorldCmd))
In the initializePlugin function, we will need to use the plugin_fn set to register HelloWorld command. Now I can use this function set to register the command using its register command method and then I need to pass in the command name which was the class level variable I created.
When registering and deregistering, error checking is essential, if the process fails it will throw an error so I can add a try and except block around this call to register command. As seen in the code above, using the try and except block to print an error if it fails to register.
Now basically you have a working command that you can use in Maya, to test just save this file and load it in your Plugin Manager in Maya. Once loaded, write "Hello World" in the MEL command line or use the Python code "cmds.HelloWorld()" and you should the output as follows.
"My First MPxCommand, Hello World!"
And just like this, you have written your first MPxCommand, you can use this template and write your own Command for Maya and have any functionality possible.
Until next time.
Comentarios