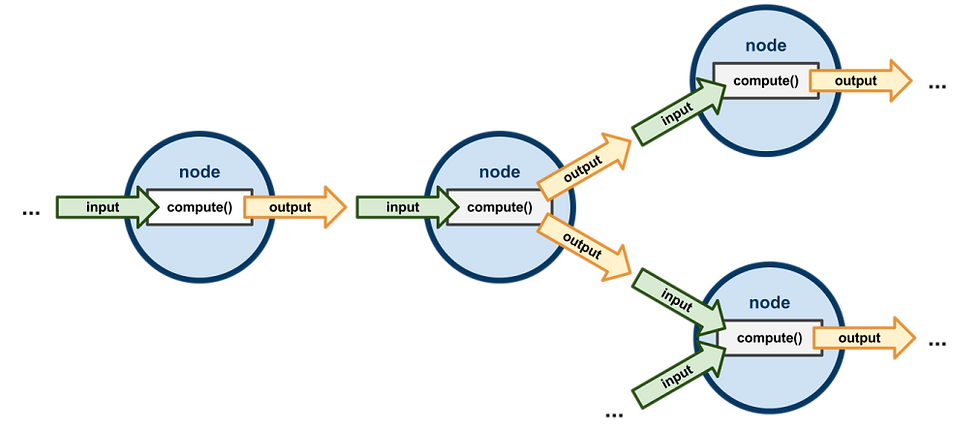
Let's try to understand how the latest version of the API and according to Autodesk not only simplifies things but also offers significant performance improvements, that being said despite being around since Maya 2012 it is still incomplete, fortunately, it is possible to use both API 1.0 and 2.0 in the same script, with some notable caveat, however, but first from a coding standpoint how do you use the different API versions and how can you identify which API version is being used in a given script, this is very easy actually you simply need to check the import statements.
# Python API 2.0
import maya.api.OpenMaya as om2
om2.MGlobal.displayInfo("Using API 2.0")
# Python API 1.0 (Legacy - only use if not supported in Python API 2.0)
# modules found in "maya" (e.g. maya.OpenMaya)
import maya.OpenMaya as om1
om1.MGlobal.displayInfo("Using API 1.0")
The Maya Python API version 2 modules are prefixed by the string Maya.API so if I want to import OpenMaya this is simply written as the code above.
As you can see from the example above, I am using the MGlobal class from both API 2.0 and API 1.0, using the function displayInfo to just print any MString text as a message.
Notice the difference between both versions, there is the difference of API module imported from Maya.
Now you may be thinking great I will always stick with Maya.API in my imports and I can avoid API 1.0 entirely, unfortunately, this is not the case as I mentioned earlier version 2.0 of the python API is incomplete and you are likely to need to fall back on version 1.0.
If care is taken this usually isn't an issue version 1.0 and version 2.0 can exist in the same script.
Here is an example:
# Python API 1.0 and 2.0 can coexist in the same script...
from PySide2 import QtWidgets
from shiboken2 import wrapInstance
# Maya Python API 2.0
from maya.api.OpenMaya import MGlobal
# Maya Python API 1.0
from maya.OpenMayaUI import MQtUtil
def maya_main_window():
"""
Return the Maya main window widget as a Python object
"""
main_window_ptr = MQtUtil.mainWindow() # Python API 1.0
return wrapInstance(long(main_window_ptr), QtWidgets.QWidget)
class ButtonDialog(QtWidgets.QDialog):
def __init__(self, parent=maya_main_window()):
super(ButtonDialog, self).__init__(parent)
button = QtWidgets.QPushButton("Hello World!", self)
button.clicked.connect(self.print_error)
def print_error(self):
MGlobal.displayError("Python API 2.0 Error Message") # Python API 2.0
if __name__ == "__main__":
try:
dialog.close() # pylint: disable=E0601
dialog.deleteLater()
except:
pass
dialog = ButtonDialog()
dialog.show()
Here I have a simple PySide2 UI which contains a single push button, when I push the button it says print error, it's printing out an error message using the MGlobal display error method, and this is using version 2.0 of the MGlobal class so you can see when I import MGlobal, it's using Maya.API so this is API version 2.0, now the UI itself needs to use the MQtUtil class to retrieve my as the main window, however, MQtUtil is not part of the version 2.0 API at least not as of Maya 2019, this means using version 1.0 is necessary, simple enough MGlobal as I just showed you is imported using Maya.API and MQtUtil is using simply Maya, meaning it's version 1.0
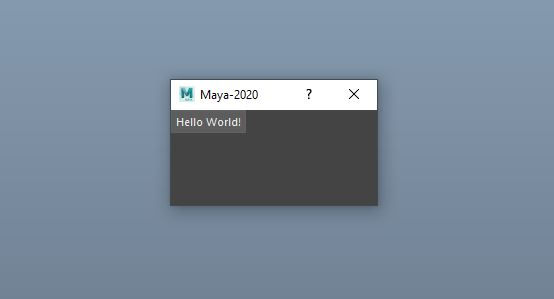
Now because objects from these two classes do not directly interact one of them is used to get Maya's main window where is the other one is simply a static method called to print out an error message, because they're never directly interacting there is no issue, that being said, here I have a simple example that duplicates part of the PolySphere Mel command, it creates a transform node a Mesh node, as well as a PolySphere node and at the very end the PolySphere node is being connected to the mesh code.
import maya.api.OpenMaya as om
# Create Transform Node
transform_fn = om.MFnDagNode()
transform_obj = transform_fn.create("transform", "pSphere1")
# Create Mesh Node
mesh_fn = om.MFnDagNode()
mesh_obj = mesh_fn.create("mesh", "pSphereShape1", transform_obj)
# Create PolySphere Node
sphere_fn = om.MFnDependencyNode()
sphere_obj = sphere_fn.create("polySphere")
# Find Sphere node's output plug
src_plug = sphere_fn.findPlug("output", False)
# Find Mesh node's inMesh plug
dest_plug = mesh_fn.findPlug("inMesh", False)
# Connect PolySphere node's output to Mesh node's input
dg_mod = om.MDGModifier()
dg_mod.connect(src_plug, dest_plug)
dg_mod.doIt()
# Get initialShadingGroup MObject
selection_list = om.MSelectionList()
selection_list.add("initialShadingGroup")
# Add mesh object to the initialShadingGroup set
set_fn = om.MFnSet(selection_list.getDependNode(0))
set_fn.addMember(mesh_obj)
Running this code creates a new PolySphere, but because the initial shading group isn't being assigned as happens in the PolySphere Mel command, right now it just shows up as a bright green object.

I would like to draw your attention to is that this script is using the Maya Python API 2.0 exclusively, the MObjects function sets and plugs are all version 2.0 objects and can interact with each other without issue, that being said, if the mesh objects were created using version 1.0, and the sphere objects were created using version 2.0, then the plugs returned, when using the findPlug method, would result in one of them being a version 1.0 plug and the other being a version 2.0 plug, and when I tried to connect them, using the MDGModifier connect method which takes 2 plug objects this would fail, if the MDGModifier object was created using version 1.0 it would expect version 1.0 plugs, if this object were created using version 2.0 it would expect version 2.0 plugs, now in a very simple example something like this is very unlikely to occur. It is when the code gets more complex and is split up between multiple files that version 1.0 now in version 2.0 objects maybe mixed leading to unexpected and difficult to identify errors, a production pipeline would be a prime example of where this could occur, multiple files created over several years by different people require special care to be taken. Even developing tools for personal use, forgetting to use Maya.API in a single file is all it takes to lead to the debugging nightmare so take a few extra seconds to verify that you are using the correct python API version.
Maya API - Undo/Redo
When using the Maya API, any operations that modify the scene much have an associated undo and redo operation.
Examples of modifying the scene:
Adding/deleting nodes
Adding/deleting attributes
Changing attribute values
Adding/removing connections
Failing to implement undo/redo operations or not implementing them correctly can result in a corrupt undo stack.
A Corrupt Undo Stack can lead to:
Unexpected crashes
Unusual and downright weird behaviour
Implementing undo/redo operations requires creating a new Maya (MEL) Command and this involves:
Extending the MPxCommand class
Implementing the logic in the undo and redo methods
Registering the new command with Maya (requires a plugin)
Yes, you need to create a plugin to implement undo/redo functionality when using the Maya API.
Until next time.
Kommentare