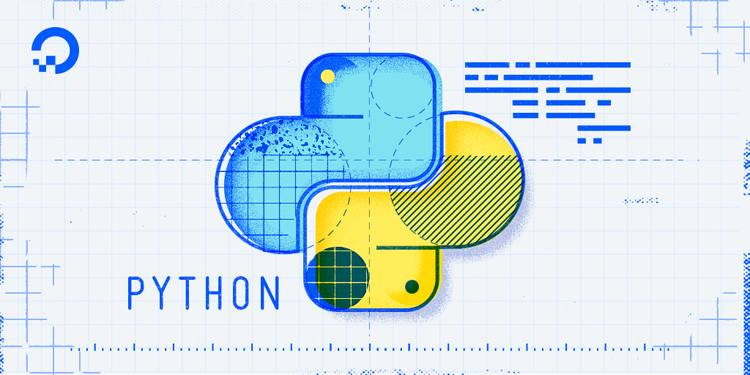
Python 3 is the latest major version of the Python programming language. It was released in 2008, and is designed to be a more robust, secure, and expressive language than Python 2.
There are many changes and updates from Python 2 to Python 3. Some of the most significant changes include:
Print statement: In Python 2, the print statement is a keyword. In Python 3, it is a function. This means that you can now use the print function in expressions, and you can also pass arguments to it.
Division: In Python 2, division of integers is performed using floor division. This means that 5 / 2 is equal to 2, not 2.5. In Python 3, division of integers is performed using floating-point division. This means that 5 / 2 is equal to 2.5.
Unicode support: Python 3 has improved Unicode support. This means that you can now use Unicode characters in your code without having to worry about encoding issues.
New features: Python 3 has a number of new features, including:
Generators: Generators are a way to create iterators. They are more efficient than lists, and they can be used to create lazy evaluation.
Context managers: Context managers are a way to manage resources. They are used to ensure that resources are closed properly, even in the event of an exception.
Type annotations: Type annotations are a way to annotate the types of variables and expressions in your code. They can be used to improve the readability and maintainability of your code.
These are just a few of the changes and updates from Python 2 to Python 3. If you are still using Python 2, I encourage you to upgrade to Python 3. It is a more modern, secure, and expressive language that will make your coding life easier.
Code Examples
Here are some code examples that demonstrate the changes from Python 2 to Python 3:
# Python 2
print 'Hello, world!'
# Python 3
print('Hello, world!')
In Python 2, the print statement is a keyword. In Python 3, it is a function. This means that you can now use the print function in expressions, and you can also pass arguments to it.
# Python 2
5 / 2 # 2
# Python 3
5 / 2 # 2.5
In Python 2, division of integers is performed using floor division. This means that 5 / 2 is equal to 2, not 2.5. In Python 3, division of integers is performed using floating-point division. This means that 5 / 2 is equal to 2.5.
# Python 2
'hello'.encode('utf-8')
# Python 3
'hello'.encode()
In Python 2, you need to specify the encoding when you encode a string. In Python 3, the encoding is automatically detected.
# Python 2
def generator():
for i in range(10):
yield i
for i in generator():
print(i)
# Python 3
def generator():
for i in range(10):
yield i
gen = generator()
for i in gen:
print(i)
Generators are a way to create iterators. They are more efficient than lists, and they can be used to create lazy evaluation.
The generator code examples are identical in Python 2 and 3, but there is a subtle difference in how they work. In Python 2, the generator function is executed immediately, and the values are yielded one at a time. In Python 3, the generator function is not executed until it is first called. This means that in Python 3, the generator function is not created until it is needed, which can improve performance.
The context manager examples are identical in Python 2 and 3, but there is a subtle difference in how they work. In Python 2, the context manager is executed immediately, and the resources are released when the with statement is exited. In Python 3, the context manager is not executed until it is first entered, and the resources are released when the with statement is exited, even if an exception is raised.
# Python 2
with open('myfile.txt') as f:
for line in f:
print(line)
In this case, the open() function is executed immediately, and the file is opened. The for loop iterates over the file, and the file is closed when the for loop is exited.
Here is the same context manager in Python 3:
# Python 3
with open('myfile.txt') as f:
try:
for line in f:
print(line)
finally:
f.close()
In this case, the open() function is not executed until it is first entered. The try block contains the code that uses the file, and the finally block contains the code that closes the file. If an exception is raised in the try block, the finally block will still be executed, and the file will be closed.
The difference between the two versions of the context manager is subtle, but it can have a significant impact on the way that errors are handled. In Python 2, if an exception is raised in the with statement, the file will not be closed. This can lead to resource leaks. In Python 3, the file will always be closed, even if an exception is raised. This makes it easier to write code that is free of resource leaks.
In general, it is recommended to use context managers in Python 3 whenever possible. Context managers help to ensure that resources are released properly, even in the event of an exception.
Conclusion
Python 3 is a major upgrade from Python 2. It is a more modern, secure, and expressive language that will make your coding life easier. If you are still using Python 2, I encourage you to upgrade to Python 3.
Here are some resources that you may find helpful in migrating your code from Python 2 to Python 3:
[Python 3 Migration Guide](https://docs.python.org/3/)
コメント